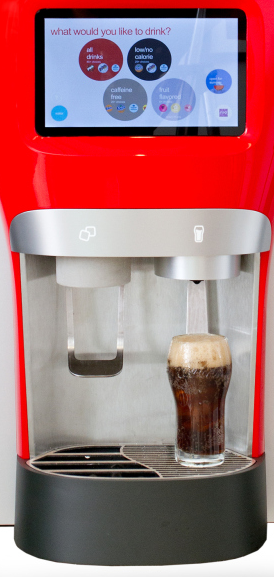
In this set of tutorials we will:
Define a dispenser that we’ll work with in our ongoing KOS application tutorials (this page)
Write Java code that contains a circuit board and pumps
Test this new code by setting a breakpoint and viewing program values
Preliminaries
You should have completed the Hello World tutorial and have it ready build upon. |
We are going to write Java code for our imaginary dispenser. It has a single display where the customer chooses his/her desired drink, a button that allows pouring of the beverage, and a single nozzle where it is dispensed. It could look something like this:
We are going to keep its beverage list simple: the customer can choose from Coke, Diet Coke, Orange Soda, Grape Soda, and water.
The dispenser has a single-board computer, a nozzle, plain and carbonated water sources, and the four syrups. The following diagram illustrates the dispenser.
The hardware includes:
Six valves, one for each ingredient
One SBC (single-board computer) that controls each valve (on or off)
One nozzle for mixing and dispensing the ingredients, making the beverage
All of these are contained inside an assembly
Here’s a sneak peek at the software components we’ll use that mimick the actual dispenser hardware:
We’ll have one Assembly that contains one Board, one Nozzle, and six Holder objects. A Holder
represents how the ingredient connects to the dispenser. Each Holder
connects to a single Pump, which controls the flow of each ingredient.
Valve vs. Pump
A valve is a special case of a pump. A valve has a simple on/off mechanism and a fixed flow rate. A pump controls and monitors the actual flow rate. The corresponding class in KOS is named Pump, so that’s the terminology we’ll use. |
Multiple hardware components
In our example we have a single board and one nozzle. However, in many dispensers the assembly contains multiple boards and/or multiple nozzles. In addition, a holder can attach an ingredient to multiple pumps. KOS supports these variations and more. These simplifications are made to reduce the complexity of this first demo dispenser example. |
In this and succeeding tutorials, we’ll write a KOS application that controls the computer, which then delivers the ingredients in the desired ratios to the nozzle, providing the customer with a cold refreshment!
On the next page, we’ll write some code to control some of these physical entities we’ve defined.